How to Use JavaScript Code in HTML Code
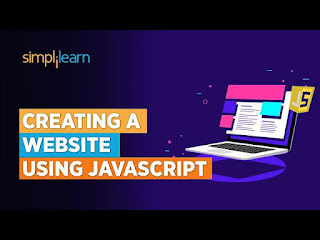.jpeg)
JavaScript is a powerful scripting language that enables developers to add interactive and dynamic elements to their web pages. Integrating JavaScript within an HTML document can enhance the user experience by making web pages more engaging and functional. This guide will walk you through how to incorporate JavaScript code within your HTML structure and explain various methods with detailed examples.
1. Inline JavaScript
Inline JavaScript is written directly within the HTML elements using the onclick
, onmouseover
, or other event attributes. This is useful for small scripts that do not need a separate script block.
<button onclick="alert('Hello, World!')">Click Me</button>
In this example, when the user clicks the button, the JavaScript code triggers an alert displaying "Hello, World!"
2. Internal JavaScript
Internal JavaScript is placed within the <script>
tags in the <head>
or <body>
section of the HTML document. This approach is useful for adding more complex JavaScript logic while keeping it within the same file as the HTML.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Internal JavaScript Example</title> <script> function greetUser() { alert('Welcome to our website!'); } </script> </head> <body> <h1>Hello, World!</h1> <button onclick="greetUser()">Greet</button> </body> </html>
In the above code, the JavaScript function greetUser()
is defined within the <script>
tag and is called when the user clicks the button.
3. External JavaScript
External JavaScript allows you to write your JavaScript code in a separate file and link it to your HTML document. This method helps keep your code organized, makes it easier to maintain, and promotes reusability.
To use an external JavaScript file, create a new file with the .js
extension (e.g., script.js
) and include it in your HTML document using the <script>
tag with the src
attribute.
// script.js function showMessage() { console.log('This is an external JavaScript file.'); }
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>External JavaScript Example</title> <script src="script.js"></script> </head> <body> <h1>Welcome</h1> <button onclick="showMessage()">Show Message</button> </body> </html>
In this setup, the showMessage()
function is defined in the script.js
file and is triggered by the button click event.
4. Placing JavaScript in the <head> vs. <body>
JavaScript code can be placed in either the <head>
or <body>
section of the HTML document. When placed in the <head>
section, the script runs before the HTML content is fully loaded, which may cause issues if the script relies on the DOM elements. For better performance, it is generally recommended to place the <script>
tag at the end of the <body>
section to ensure that the HTML content loads first.
<body> <h1>Hello, World!</h1> <button onclick="greetUser()">Greet</button> <script> function greetUser() { alert('Hello after the content loads!'); } </script> </body>
5. Using JavaScript for Interactivity
JavaScript allows developers to create interactive experiences for users. Examples include form validation, real-time updates, and dynamic content manipulation. Here's a simple form validation example:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Form Validation</title> </head> <body> <form onsubmit="return validateForm()"> <label for="name">Name:</label> <input type="text" id="name" name="name"> <input type="submit" value="Submit"> </form> <script> function validateForm() { var name = document.getElementById('name').value; if (name == '') { alert('Name must be filled out'); return false; } return true; } </script> </body> </html>
This script checks whether the name input field is empty when the form is submitted. If it is empty, an alert is displayed, and the form submission is prevented.
By using JavaScript within your HTML code in these different ways, you can build interactive and user-friendly web pages that respond to user actions effectively.